说实话没接触 Apache ant 挺长时间了,几乎被我忘记在了某个角落。
今天在某一个项目中发现。
有个配置文件,每次在发布打包的时候都需要改成生产配置,然而本地DEBUG和开发的时候又需要改成本地设置。
这个操作从工作量上说,确实没啥,但时间长了就觉得太麻烦了。这种事情我是比较忍不了的。
这个项目目前是用 ANT
构建的,那么我们当然可以使用 ANT
来完成我们需要的属性替换。
搭建ANT工程
为了测试,我们搭建一个简单的 ANT
工程。
首先准备一个空目录,创建一个叫 build.xml
的文件,内容如下:
1 2 3 4 5 6 7
| <project name="MyProject" default="global" basedir="."> <target name="print"> <echo>Replacement by ANT, test!</echo> </target>
<target name="global" depends="print"/> </project>
|
保存文件后,在 cmd
命令行执行 ant
显示如下表示成功:
1 2 3 4 5 6 7 8 9
| Buildfile: xxxxx\build.xml
print: [echo] Replacement by ANT, test!
global:
BUILD SUCCESSFUL Total time: 0 seconds
|
一般的字符替换
准备一个待被替换的文本,比如下面这样,我起名为 user.json
:
1 2 3 4 5 6 7
| { "name": "@name@", "sex": "@sex@", "height": "@height@", "weight": "@weight@", "address": "@address@" }
|
保存后,在 build.xml
中修改为如下的内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <project name="MyProject" default="global" basedir="."> <target name="print"> <echo>Replacement by ANT, test!</echo> </target>
<target name="replace"> <replace file="user.json" token="@name@" value="Jeremy"/> <replace file="user.json"> <replacetoken>@sex@</replacetoken> <replacevalue>male</replacevalue> </replace> </target>
<target name="global" depends="print,replace"/> </project>
|
保存文件后,在 cmd
命令行执行 ant
显示如下表示成功:
1 2 3 4 5 6 7 8 9 10 11
| Buildfile: xxxxx\build.xml
print: [echo] Replacement by ANT, test!
replace:
global:
BUILD SUCCESSFUL Total time: 0 seconds
|
同时, user.json
已经修改成了如下的内容:
1 2 3 4 5 6 7
| { "name": "Jeremy", "sex": "male", "height": "@height@", "weight": "@weight@", "address": "@address@" }
|
一般这样就可以完成指定的字符串替换了。但是 ANT
还支持一种引用属性文件来替换字符的办法,下面看例子。
使用properties文件进行字符替换
首先,准备属性文件 values.properties
,内容如下:
1 2 3 4 5
| name=Jeremy sex=male height=175 weight=69 address=Xi'an, China
|
同时,修改 build.xml
为如下内容:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <project name="MyProject" default="global" basedir="."> <target name="print"> <echo>Replacement by ANT, test!</echo> </target>
<target name="replace"> <replace file="user.json" propertyfile="values.properties"> <replacefilter token="@name@" value="Lucy"/> <replacefilter token="@sex@" value="female"/> <replacefilter token="@height@" property="height"/> <replacefilter token="@weight@" property="weight"/> <replacefilter token="@address@" property="address"/> </replace> </target>
<target name="global" depends="print,replace"/> </project>
|
记得将 user.json
还原为如下内容:
1 2 3 4 5 6 7
| { "name": "@name@", "sex": "@sex@", "height": "@height@", "weight": "@weight@", "address": "@address@" }
|
在 cmd
命令行执行 ant
后,user.json
中的内容将会变更成如下的样子:
1 2 3 4 5 6 7
| { "name": "Lucy", "sex": "female", "height": "175", "weight": "69", "address": "Xi'an, China" }
|
这样完成了使用 property 文件进行字符替换,其中需要注意的是上文的 build.xml
中标注了 ① 和 ②的位置。
这两种写法是不一样的,仅仅是第二种写法使用了 property 文件的属性。
除过上面介绍到的方法之外,ANT
也支持使用正则来匹配要替换的内容,接着往下看。
使用正则表达式匹配待替换字符
使用正则表达式匹配替换字符的简单语法如下:
1 2 3 4
| <replaceregexp file="${src}/build.properties" match="OldProperty=(.*)" replace="NewProperty=\1" byline="true"/>
|
其中:
- file: 表示待修改的文件
- match: 匹配的正则表达式
- replace: 需要替换后的内容
- byline: 表示替换是否逐行匹配。默认为
false
,表示将整个文本当成一个字符串来处理。
继续用上面的例子,先将 user.json
还原为如下:
1 2 3 4 5 6 7
| { "name": "@name@", "sex": "@sex@", "height": "@height@", "weight": "@weight@", "address": "@address@" }
|
修改 build.xml
的内容为:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <project name="MyProject" default="global" basedir="."> <target name="print"> <echo>Replacement by ANT, test!</echo> </target>
<target name="replace"> <replaceregexp file="user.json" match="@.*@" replace="unknown" byline="true"/> </target>
<target name="global" depends="print,replace"/> </project>
|
同样,在 cmd
命令行执行 ant
, 此时 user.json
的内容将会被变更为:
1 2 3 4 5 6 7
| { "name": "unknown", "sex": "unknown", "height": "unknown", "weight": "unknown", "address": "unknown" }
|
完整示例
此处将本篇文章用到的 replace 方法集中在一处,以便进行参考。
当然具体项目还要根据实际情况来编写,下面的这种后面的 replace 肯定是不会生效的,因为前面已经被替换完了。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| <project name="MyProject" default="global" basedir="."> <target name="print"> <echo>Replacement by ANT, test!</echo> </target>
<target name="replace"> <replace file="user.json" token="@name@" value="Jeremy"/> <replace file="user.json"> <replacetoken>@sex@</replacetoken> <replacevalue>male</replacevalue> </replace>
<replace file="user.json" propertyfile="values.properties"> <replacefilter token="@name@" value="Lucy"/> <replacefilter token="@sex@" value="female"/> <replacefilter token="@height@" property="height"/> <replacefilter token="@weight@" property="weight"/> <replacefilter token="@address@" property="address"/> </replace>
<replaceregexp file="user.json" match="@.*@" replace="unknown" byline="true"/> </target>
<target name="global" depends="print,replace"/> </project>
|
参考
欢迎关注我的公众号 须弥零一,跟我一起学习IT知识。
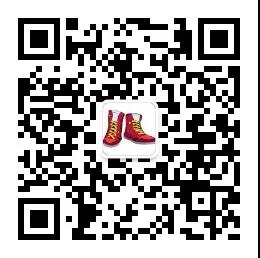
如果您喜欢此博客或发现它对您有用,则欢迎对此发表评论。 也欢迎您共享此博客,以便更多人可以参与。 如果博客中使用的图像侵犯了您的版权,请与作者联系以将其删除。 谢谢 !